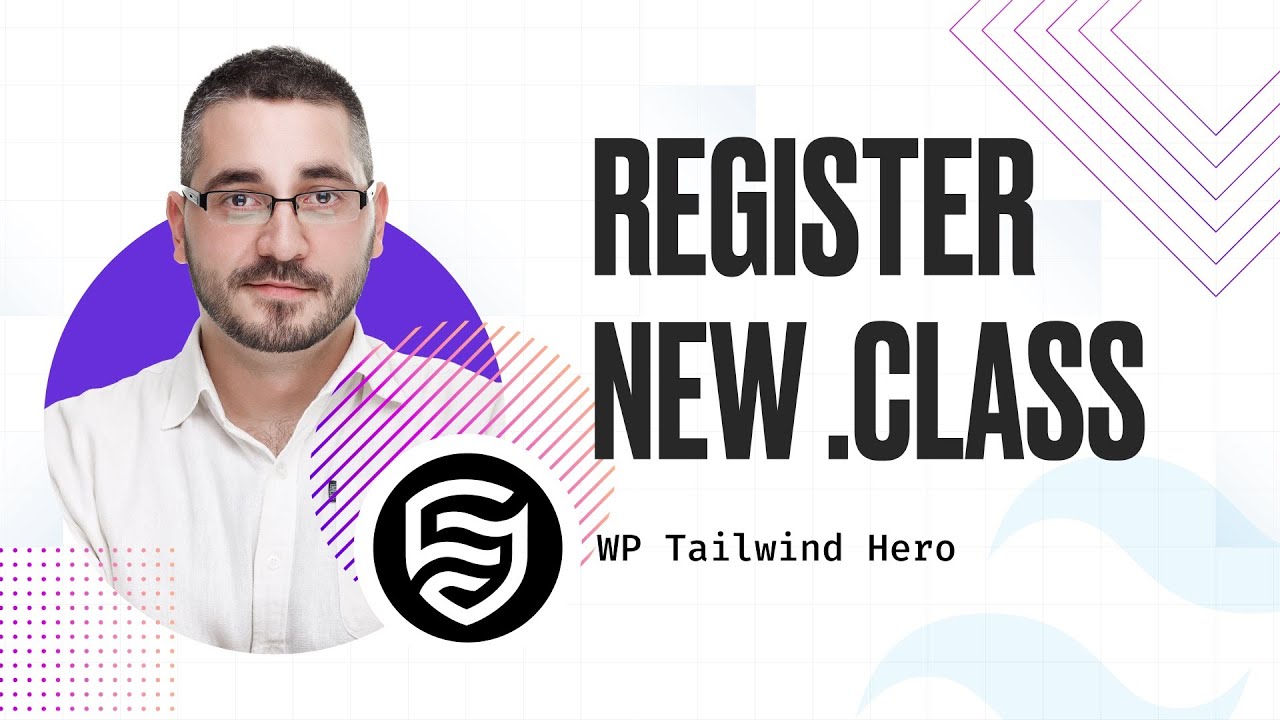
Hello and welcome! Today, we’re going to explore adding new classes in Tailwind CSS, which, like all frameworks, isn’t without its imperfections. Our goal is to create a custom class that we can reuse throughout our project.
To begin, navigate to the Tailwind CSS official website and access the documentation. Here, you’ll want to look for the “Add Components” section. As you scroll down, you’ll find various examples that can guide us through the process.
Let’s start by cleaning up the default settings. We’ll need to modify the Tailwind configuration, which requires the use of the plugin function. Copy the necessary code from the plugins section, and remember, using module.exports
works just like a default export.
Here’s where it gets interesting: we’re adding a new class named BTN
. This class includes properties like padding, border radius, and font weight, which are crucial for styling. Once we register this class, typing BTN
will prompt Tailwind to autocomplete it, allowing for quick implementation in your HTML.
const plugin = require('tailwindcss/plugin')
export default {
plugins: [
plugin(function({ addComponents }) {
addComponents({
'.btn': {
padding: '.5rem 1rem !important',
borderRadius: '.25rem !important',
fontWeight: '600 !important',
backgroundColor: #ff3300,
},
// ...
})
})
]
}
If you’re looking to customize further, for instance adding a background color using Tailwind’s color palette, you’d type theme.colors.red.300
. This approach lets you extend values directly from Tailwind without manually coding each property.
const plugin = require('tailwindcss/plugin')
export default {
plugins: [
plugin(function({ addComponents }) {
addComponents({
'.btn': {
padding: '.5rem 1rem !important',
borderRadius: '.25rem !important',
fontWeight: '600 !important',
backgroundColor: theme(colors.red.300)
},
// ...
})
})
]
}
You might wonder why not just write traditional CSS and use apply
for background styles. The reason is simple: this method doesn’t update Tailwind’s autocomplete features, meaning Tailwind won’t recognize these custom classes by default.
To make these classes appear in Tailwind’s autocomplete suggestions, you need to integrate them into the configuration as shown. Let’s say you choose a background color of red.200
. Once integrated, it appears immediately in the autocomplete suggestions, making it super efficient.
For those unfamiliar with converting CSS properties to JavaScript, numerous online tools can assist you. Simply search for “CSS to JavaScript” in your preferred search engine to find these resources. They can transform your standard CSS into JavaScript objects, which you can then incorporate directly into your Tailwind setup.
By the end of this session, you’ll see how simple it is to create custom classes in Tailwind CSS. You can copy and paste these classes directly from your JavaScript file, ensuring consistency across your project.
Resources
Thank you for joining today’s tutorial. If you have any suggestions or comments, please leave them below to help us improve our future tutorials. See you next time, and happy coding!